Static-site generation integration with Flagship [SSG]
This guide explains you how to use Flagship with nextJS using SSG mode.
How it works
In Static-site generation, content is rendered at build time during which we will fetch all flags targeting a unique flagship visitor and pass them (flags) through a props to the __app.js
component to feed the FlagshipProvider
component.
Notes
- All your visitors will see the same flags because at build time we can only target one visitor.
- If you update your campaigns from Flagship dashboard, you will also need to rebuild your app to update your static content. Check
- Once your app is loaded on a browser, you can use Flagship SDK the same way as in client side rendering mode
Implementation
Please refer to this article link to setup a NextJS project.
Once your project is set up, you need to install and initialize the Flagship React SDK.
yarn add @flagship.io/react-sdk
We will customize the next component App to initialize the Flagship SDK.
// pages/_app.jsx
import { FlagshipProvider } from '@flagship.io/react-sdk'
import '../styles/globals.css'
function MyApp({ Component, pageProps }) {
const { initialVisitorData, flagsData } = pageProps
return (
<FlagshipProvider
envId={process.env.NEXT_PUBLIC_ENV_ID}
apiKey={process.env.NEXT_PUBLIC_API_KEY}
visitorData={initialVisitorData || {}}
initialFlagsData={flagsData}
>
<Component {...pageProps} />
</FlagshipProvider> )
}
export default MyApp
We have 2 props in MyApp
component, initialVisitorData
and initialFlagsData
. the first one contains visitor data and the second contains the FlagsData fetched from getStaticProps
at build time to feed FlagshipProvider
.
Here is the index page:
//pages/index.jsx
import {
useFsFlag,
useFlagship,
Flagship,
HitType,
EventCategory,
} from "@flagship.io/react-sdk";
import styles from "../styles/Home.module.css";
export default function Home() {
const fs = useFlagship();
//get flag
const myFlag = useFsFlag("my_flag_key");
const onSendHitClick = () => {
fs.sendHits({
type: HitType.EVENT,
category: EventCategory.ACTION_TRACKING,
action: "click button",
});
};
return (
<div className={styles.container}>
<main className={styles.main}>
<h1>Next Static site generation integration with Flagship [SSG]</h1>
<p>flag key: my_flag_key</p>
<p>flag value: {myFlag.getValue("default-value")}</p>
<button
style={{ width: 100, height: 50 }}
onClick={() => {
onSendHitClick();
}}
>
Send hits
</button>
</main>
</div>
);
}
// This function runs only at build time
export async function getStaticProps() {
//Start the Flagship SDK
const flagship = await Flagship.start(
process.env.NEXT_PUBLIC_ENV_ID,
process.env.NEXT_PUBLIC_API_KEY,
{
fetchNow: false,
}
);
const initialVisitorData = {
id: "my_visitor_id",
context: {
any: "value",
},
};
// Create a new visitor
const visitor = flagship.newVisitor({
visitorId: initialVisitorData.id,
context: initialVisitorData.context,
hasConsented: true,
});
// //Fetch flags
await visitor.fetchFlags();
// Pass data to the page via props
return {
props: {
initialFlagsData: visitor.getFlags().toJSON(),
initialVisitorData,
},
};
}
On the getStaticProps
of the index component page, we :
- Fetch the flags for the targeted visitor
- Pass the fetched flags to the page via props
How to automate rebuilding
To automate the rebuilding of your application, you need to use Flagship’s Webhooks Integrations. When your campaigns are updated in Flagship, a webhook called [environment] synchronized will be triggered and you can use this event to trigger a rebuild of your application to ensure that it reflects the updated flag values.
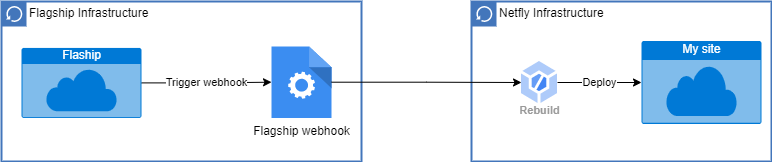
In this guide, we will deploy our app to Netlify.com, but the process is similar for other platforms and can also be used with a CI/CD pipeline.
We will proceed in two steps:
1. Once your app is deployed to netlify.com, go to Site Settings -> Build and Deploy and create a build hook.
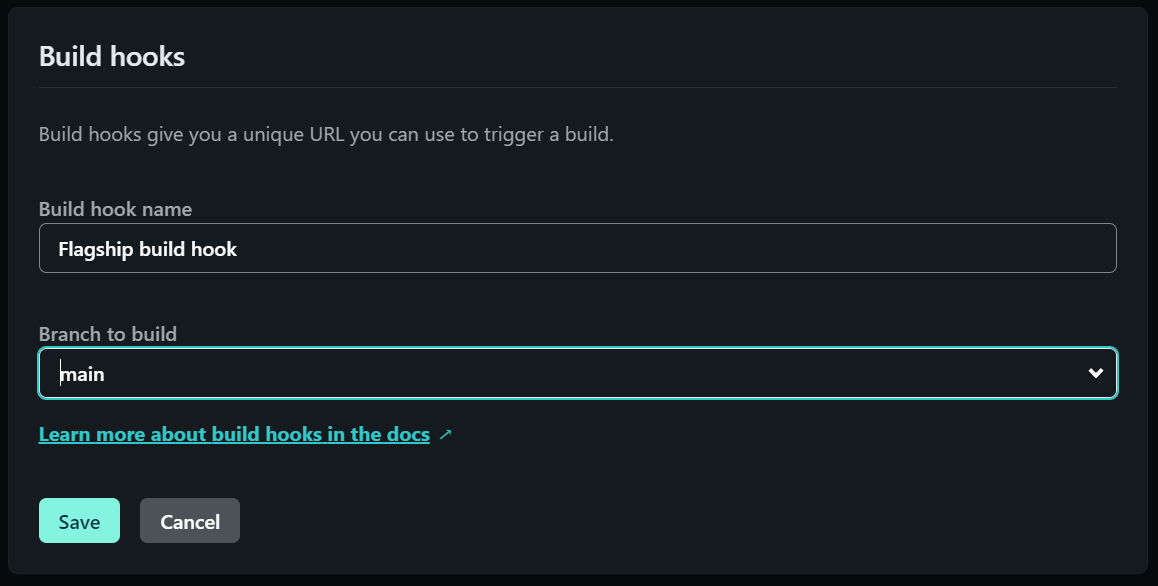
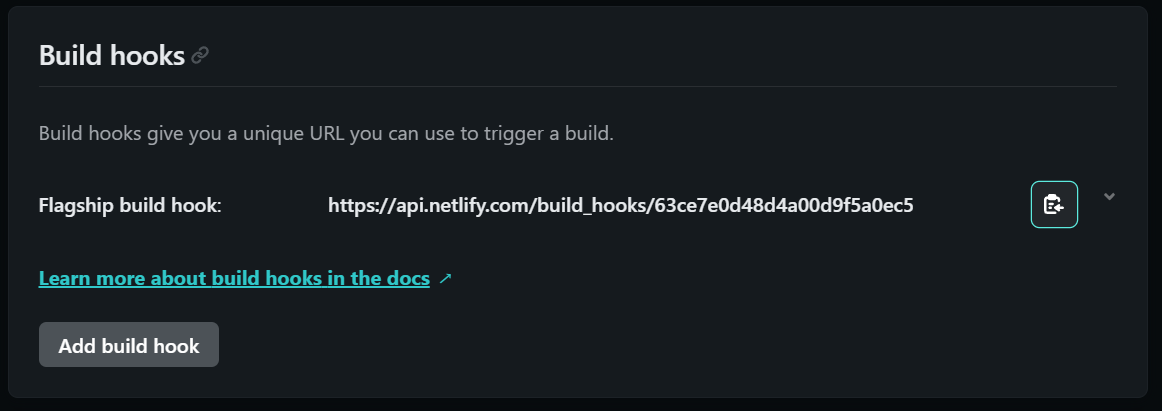
2. Set up the webhook on the Flagship platform
On the Flagship platform, go to Settings -> Integrations and select the Webhooks tab. Choose the [environment] synchronized event and enter the URL for your Netlify build hook. Then click “Create” to set up the webhook.
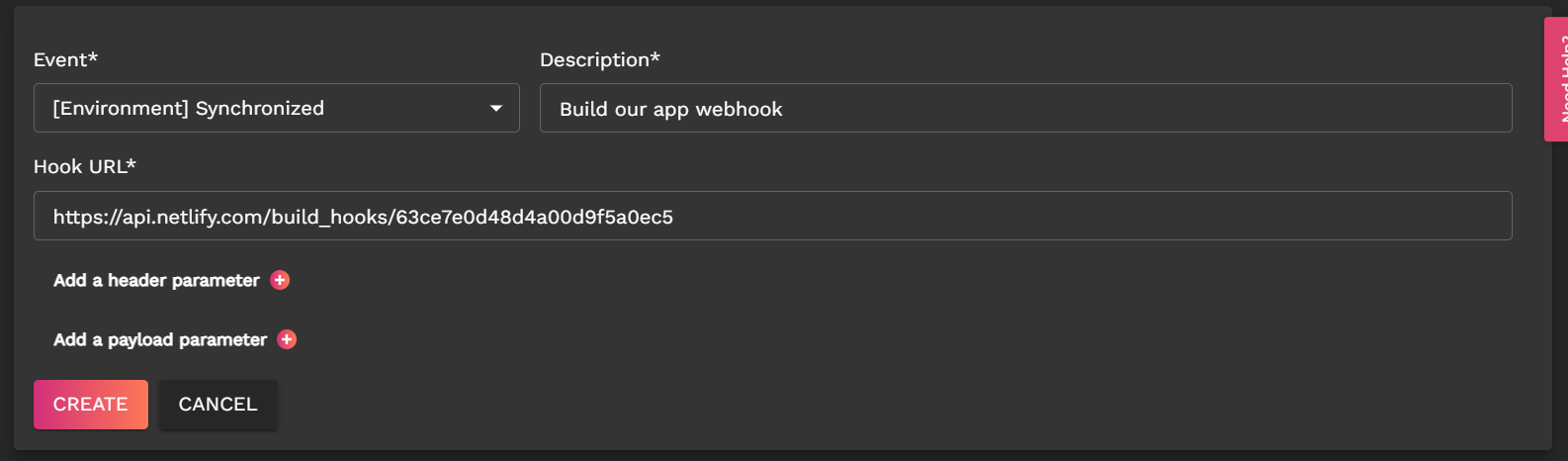
Any feedback?
Want another tutorial?
Contact us
See full code here : github
Updated 28 days ago